How to type a React form onSubmit handler
Excellent TypeScript definitions for your React forms
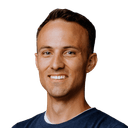
Ricky Hanlon posted on Bluesky:
what's wrong with this code:
if (props.wrap) {return <Wrapper>{children}</Wrapper>}return children
A lot of people didn't get it right away. In fact, it took me a moment thinking about it as well, but then I replied:
Does it have to do with what happens when the wrap prop changes?
He said "Yes" and I knew for sure what he was talking about.
Have you ever wondered why your React component's state sometimes disappears or resets unexpectedly? Or why two identical components don't share state? React's approach to state is all about where a component sits in the UI tree, not just what it is. This means that state is preserved as long as the same component is rendered at the same position—but if you swap components, change their keys, or move them around, React may reset their state.
A common pitfall: toggling between two components (like two players in a scoreboard) using different keys or types will reset their state every time you switch. This is often not what you want, especially if you expect the UI to "remember" previous input or progress. The React docs show how this can trip you up, and why relying on position and keys for state can be sub-optimal if you're not careful.
If you've ever been surprised by disappearing form input or state that doesn't persist the way you expect, this article is for you. It covers:
Curious to learn more and see code examples? Check out the full article: Preserving and Resetting State – React Docs
For Ricky's example specifically, if you want to preserve state while toggling
the wrapper, you need to ensure the component tree structure remains stable. One
way is to always render the Wrapper
, but conditionally apply styling or
behavior:
return ( <Wrapper style={props.wrap ? {} : { display: 'contents' }}> {children} </Wrapper>)
Or, if you want to avoid an extra DOM node when not wrapping, you can use a key to intentionally reset state, or lift state up to a parent component so it isn't lost when the tree changes. The key is to avoid swapping between two different component trees at the same position if you want to preserve state.
But really, you should just read the whole article.
Delivered straight to your inbox.
Excellent TypeScript definitions for your React forms
It wasn't a library. It was the way I was thinking about and defining state.
Whether you're brand new to building dynamic web applications or you've been working with React for a long time, let's contextualize the most widely used UI framework in the world: React.