Importing React Through the Ages
How and why I import react using a namespace
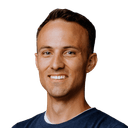
When building React applications, you'll often work with data that only exists on the client—such as items users add to a list before saving them to a server. These objects usually don't have a unique ID yet, but you still need one for things like React keys, form field associations, or other DOM relationships.
Unique IDs are essential for:
If your data comes from a backend, it often already has an ID. But for client-only data, you need to generate one yourself.
Don't generate a new ID on every render! Instead, generate the ID once—when the object is created—and store it on the object. This ensures the ID stays stable and unique. You don't necessarily need to use that ID if you end up saving that object in a database or something, but to help React keep track of the items in the list, you need to have a unique ID for each item.
The Web Crypto API provides a simple and reliable way to generate unique IDs:
crypto.randomUUID()
This function returns a universally unique identifier (UUID) string, which is perfect for most use cases.
Here's a React component that lets users add items to a list. Each item gets a unique ID when it's created:
import React, { useState } from 'react'function generateId() { return crypto.randomUUID()}function ItemList() { const [items, setItems] = useState([]) const [input, setInput] = useState('') function handleAdd() { if (!input.trim()) return setItems([...items, { id: generateId(), label: input }]) setInput('') } return ( <div> <input value={input} onChange={(e) => setInput(e.target.value)} placeholder="Add an item" /> <button onClick={handleAdd}>Add</button> <ul> {items.map((item) => ( <li key={item.id}>{item.label}</li> ))} </ul> </div> )}
When working with client-only data, it's important to generate unique IDs at the
time you create your objects, rather than generating them on every render. The
Web Crypto API's crypto.randomUUID()
function provides a reliable way to
generate these unique identifiers. Make sure to store the ID directly on the
object when you create it - this ensures the ID remains stable and can be safely
used for React keys and DOM relationships.
For more on accessibility and unique IDs, check out the related article on generating unique IDs in React.
Also check out Why React Needs a Key Prop for more on why you need to use a key prop.
Delivered straight to your inbox.
How and why I import react using a namespace
Truly maintainable, flexible, simple, and reusable components require more thought than: "I need it to do this differently, so I'll accept a new prop for that". Seasoned React developers know this leads to nothing but pain and frustration for both the maintainer and user of the component.
Build flexible, declarative UIs like native HTML with Compound Components—a powerful React pattern you’ll master at EpicReact.dev.
Control Props let you hand over component state to your users—just like a controlled input. Learn to build ultra-flexible UIs at EpicReact.dev.