Improve the Performance of your React Forms
Forms can get slow pretty fast. Let's explore how state colocation can keep our React forms fast.
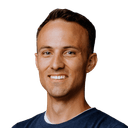
If you've ever used a native <select>
and <option>
in HTML, you've already
experienced the power of compound components—even if you didn't know the
name.
But what if you could bring that same flexibility and declarative power to your own React components? That's what the Compound Components pattern is all about.
Compound components are a set of components that work together to form a complete UI, implicitly sharing state and behavior. Think of them as a family: each member has a role, but they all work together seamlessly.
The classic example is HTML's select:
<select> <option value="1">Option 1</option> <option value="2">Option 2</option></select>
The <select>
manages the state, and the <option>
s are just configuration.
You don't have to wire them up manually—they just work.
You could build a custom select like this:
<CustomSelect options={[ { value: '1', display: 'Option 1' }, { value: '2', display: 'Option 2' }, ]}/>
But what if you want to add custom attributes to an option, or change how it's displayed when selected? You'd have to keep adding more props and configuration, making your API more complex and less flexible.
Compound components let you keep your API simple and declarative:
<CustomSelect> <CustomOption value="1">Option 1</CustomOption> <CustomOption value="2">Option 2</CustomOption></CustomSelect>
Now, you can pass anything as children, and each option can be as flexible as you need.
The parent component (like <CustomSelect>
) manages the shared state and passes
it down to its children using React's context API. The children (like
<CustomOption>
) consume that context and interact with the parent as needed.
This pattern lets you build components that are:
This isn't just a theoretical pattern. It's used in some of the most popular UI libraries, like Radix UI Tabs and Radix UI Accordion. In fact, most of Radix UI is built on this pattern.
Reach for compound components when:
Want to master patterns like this—and many more? EpicReact.dev is packed with hands-on workshops, real-world examples, and deep dives into the patterns that make React apps scalable and maintainable. Whether you're a beginner or a seasoned pro, you'll find something to level up your React skills.
Delivered straight to your inbox.
Forms can get slow pretty fast. Let's explore how state colocation can keep our React forms fast.
If you use a ref in your effect callback, shouldn't it be included in the dependencies? Why refs are a special exception to the rule!
How and why I import react using a namespace
The sneaky, surreptitious bug that React saved us from by using closures.